Liquid variables in Shopify Flow
Variables are placeholders that are replaced with values when a workflow runs. These variables describe the attributes of the customers, orders, and products that are involved in your workflows. For example, there are variables for the order number, order price, customer name, and so on. Variables can be used in conditions to control the logic in your workflow, or to output data in an action.
On this page
About Liquid
Liquid is a template language that is used to access variables in actions and to write code in Flow. Flow uses a variant of Liquid that most closely follows the open source library. Shopify themes use another variant of Liquid, but this variant of Liquid is specific to themes and includes many more filters and tags than Flow supports, as well as a different syntax for accessing variables.
Liquid variables
You can add Liquid variables to any text field that contains the Add a variable link. Click the Add a variable link beneath the relevant field, and then choose a variable from the list.
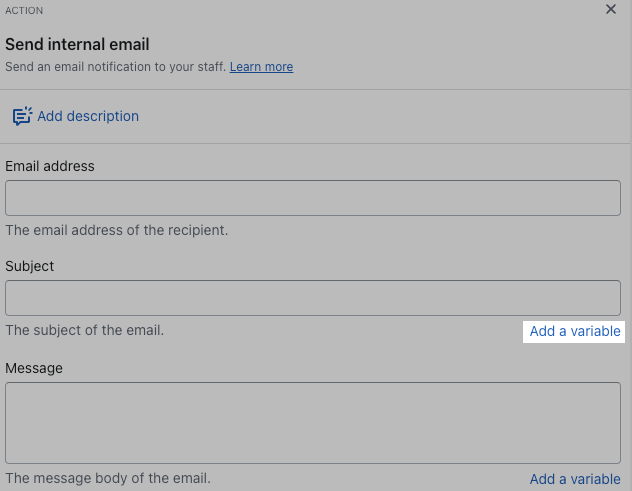
The variables in the Add a variable list are filtered, so that you only see the variables return by steps prior to the current step, such as triggers. For example, the Order Created trigger provides order and shop resources, which then allow you to use any variables related to the order or your shop settings which are accessible in the GraphQL Admin API. After you choose a variable from the list, it's properly formatted and added to the text box for you.
You can also write the liquid directly in the text block. For example, you can use the variable {{ order.name }}
to display the order string shown in the Shopify admin, such as order-123.
Because Flow uses the GraphQL Admin API to retrieve data that you use in Liquid, the variable syntax uses "camel case". For example, to access the date a product was created, enter {{ product.createdAt }}
. If you use the Liquid syntax in a Shopify theme, then you would enter {{ product.created_at }}
.
Conditional and iteration Liquid tags
Sometimes you might want to use Liquid tags to do the following:
- Write conditional statements, such as determining if an order total is greater than $100
- Iterate through a list of objects, such as outputting data for each line item in an order
You can use Liquid tags to write these statements and iterate through objects.
For example, the following Liquid displays the order number if the order total is greater than $100:
{% if order.totalPriceSet.shopMoney.amount > 100 %}
Order number: {{ order.name }}
{% endif %}
You can also use a for loop to iterate through a list of objects, such as line items for an order. For example, the following Liquid displays the name of each line item in an order:
{% for li in order.lineItems %}
{{ li.title }}
{% endfor %}
Flow supports the following Liquid conditional (or control flow) tags:
- if
- unless
- elsif/else
- case/when
- and/or (multiple conditions)
Flow also supports the following Liquid iteration tags:
Filters
Filters enable you to transform data in Liquid. Flow supports all of the open source Liquid filters.
For example, the following Liquid removes a prefix from an order name and outputs what is left: {{ order.name | remove: "Order-" }}
In addition to the standard Liquid filters, Flow provides date filters to get a date relative to another date to support the Scheduled time trigger and Get data features. These filters are: date_minus
and date_plus
.
To return a date one day in the future:
{{ "now" | date_plus: "1 day" }}
To return a date one day in the past:
{{ "now" | date_minus: "1 day" }}
These filters accept second
, minute
, day
, week
, month
, and year
as the duration unit, both singular (such as second
) and plural (such as seconds
). In addition to this format, you can also supply an integer (number of seconds). For example:
{{ "now" | date_minus: 3600 }}
You can also supply an ISO8601 duration string, where P1Y2D
means 1 year and 2 days:
{{ "now" | date_minus: "P1Y2D" }}
Considerations for using Liquid variables in filters
- Flow does not support dot notation that is available for some filters. For example, Flow supports
{{ order.lineItems | size }}
but not{{ order.lineItems.size }}
. - Flow does not support dot notation for metafields. For example you cannot use
{{ order.metafields.custom.hold_note }}
. Instead, you must loop over the metafields as outlined in the examples. - Flow does not support using indexes to access items in a list. For example, you cannot use
{{ order.lineItems[0].title }}
. Instead, you must loop over the line items as outlined in the examples.
Examples
To get a better sense of how to use Liquid variables, consider the following examples.
Output a metafield value
You want to output the value of an order metafield in an email. The metafield is a string and has a namespace of custom
and a key of hold_note
. The value of this metafield is Please wait to deliver this order until April 1.
. You create a workflow using the Order created trigger, and use the Send internal email action. In the Message section of the Send internal email action, you use the following variables.
Input | Output |
---|---|
{% assign hold_note = order.metafields | where: "namespace", "custom" | where: "key", "hold_note" | first %} The order has a hold note of: {{ hold_note.value }} | The order has a hold note of: Please wait to deliver this order until April 1. |
Convert a list of tags to a metafield
You want to convert a set of tags to a metafield that is a list of single-line text fields. You create a workflow using the Product added to store trigger, and use the Update product metafield action. In the Value section of the Update product metafield action, you add the following liquid code. This example assumes that you only need to set the values once when the product is created, and that the product has two relevant tags: color:red
and color:orange
.
Input | Output |
---|---|
{% capture mf_value %} {%- for tags_item in product.tags -%} {%- if tags_item contains "color:" -%} "{{- tags_item | remove_first: "color:" | strip -}}", {%- endif -%} {%- endfor -%} {% endcapture -%} [{{mf_value | remove_last: ","}}] | ["red","orange"] |
Write a dynamic email message for an order
You want to create a workflow to send an email to an employee when a customer spends more than $500 on an order. You create a workflow using the Order created trigger, set a condition that is true if the order total is above $500, and use the Send internal email action. In the Message section of the Send internal email action, you use the following variables.
Input | Output |
---|---|
Please send a personal thank you note to {{ order.customer.firstName }} {{ order.customer.lastName }}({{ order.customer.email }}) for placing an order for $ {{ order.totalPriceSet.shopMoney.amount }}. | Please send a personal thank you note to Jeanne Dupont (jeanne@example.com) for placing an order for $763.42. |
Write a dynamic email message for a product that is low in stock
You decide that you need to inform a staff member when product inventory is getting low and an order needs to be placed for more stock. You create a workflow that starts with the Inventory quantity changed trigger, and set a condition that is true if the inventory quantity prior is less than or equal to 10. In the Message section of the Send internal email action, you use the following variables.
Input | Output |
---|---|
Please reorder {{ product.title }}. Email owner@store.com to verify that they've received the purchase order. | Please reorder High Waist Leggings - Black. Email owner@example.com to verify that they've received the purchase order. |
Write a dynamic email message to notify staff about a fraudulent order
You want to cancel orders that have a high risk level, but prefer for your staff to manually cancel the order. You create a workflow that starts with the Order created trigger, and set a condition that is true if the risk level of the order is equal to high. In the Message section of the Send internal email action, you use the following variables.
Input | Output |
---|---|
Our Shopify store has received an order with a high risk of fraud. We would like to cancel this order right away, before it is sent to production: {{ order.name }} {{ order.billingAddress.lastName }}, {{ order.billingAddress.firstName }} {{ order.email }} Please confirm the new order status. Thanks! | Our Shopify store has received an order with a high risk of fraud. We would like to cancel this order right away, before it is sent to production: #1001 Dupont, Jeanne jeanne@example.com Please confirm the new order status. Thanks! |
Output the line items for an order using a for loop
When an order is received, it can be useful to send a message that contains the products ordered. You can do this by using for loop
, which repeatedly execute a block of code. Text fields that support variables also support for loops and the forloop object.
For example, you want to create a workflow that returns a list of all SKUs and quantities in an order. In the Message section of the Send internal email action, you use the following variables.
Input | Output |
---|---|
Order summary: {% for a in order.lineItems %} SKU: {{a.sku}} ( {{a.quantity}} ), {% endfor %} | Order summary: 8987097979 (50) 8877778887 (3) 888998898B (1) |
Output the line items for an order using a for loop with additional information
You decide to add more information to the email, including the product name, SKUs, price per item, and the customer's shipping information. In the Message section of the Send internal email action, you use the following variables.
Input | Output |
---|---|
Order summary: {% for a in order.lineItems %} Product: {{a.title}} SKU: {{a.sku}} Price (per unit): $ {{a.originalUnitPriceSet.shopMoney.amount}} Quantity: {{a.quantity}} {% endfor %} | Order summary: Product: High Waist Leggings - Black SKU: 8987097979 Price (per unit): $8.49 Quantity: 5 Product: Athletic Socks - Blue SKU: 888998898B Price (per unit): $5.61 Quantity: 2 |
Output some line items by combining a for loop with an if statement
You need to track items sold that are supplied by a specific vendor. In the Message section of the Send internal email action, you use the following variables and include an if
statement in your for loop
.
Input | Output |
---|---|
Acme product sold: {% for x in order.lineItems %} {% if x.vendor == 'acme-vendor' %} Product name: {{x.title}} SKU: {{x.sku}} {% endif %} {% endfor %} | Acme product sold: Product name: High Waist Leggings - Black SKU: 8987097979 |
Complex data objects in Shopify Flow
Flow allows you to access nearly any data that is in the GraphQL Admin API This includes complex data objects, such as lists and objects. However, there are some limitations to what you can do with these objects. The section outlines these limitations and provides examples of how to work with them.
Instead of calling lists and objects directly, you should loop over list and include only the fields that you want.
For example, instead of calling {{ order.lineItems }}
directly, use the following format to call specific fields. These examples include all the fields that would be included by calling the list or object directly. Copy and paste the fields that you need.